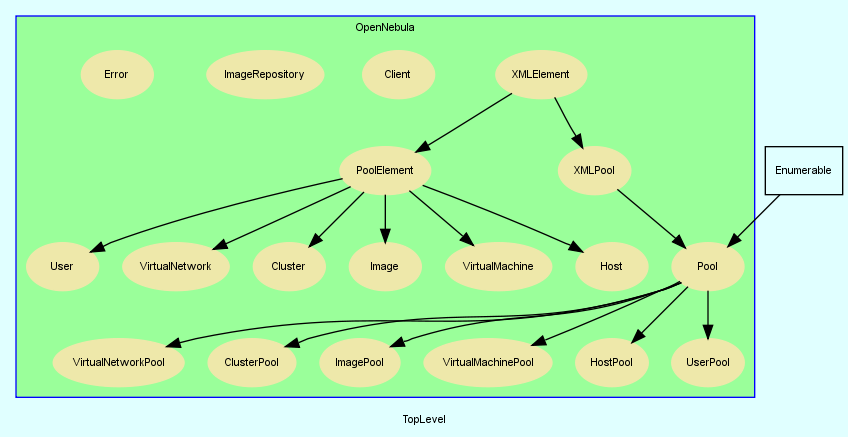
Methods
public class
public instance
Constants
VM_METHODS | = | { :info => "vm.info", :allocate => "vm.allocate", :action => "vm.action", :migrate => "vm.migrate", :deploy => "vm.deploy", :savedisk => "vm.savedisk" } | Constants and Class Methods | |
VM_STATE | = | %w{INIT PENDING HOLD ACTIVE STOPPED SUSPENDED DONE FAILED} | ||
LCM_STATE | = | %w{LCM_INIT PROLOG BOOT RUNNING MIGRATE SAVE_STOP SAVE_SUSPEND SAVE_MIGRATE PROLOG_MIGRATE PROLOG_RESUME EPILOG_STOP EPILOG SHUTDOWN CANCEL FAILURE CLEANUP UNKNOWN} | ||
SHORT_VM_STATES | = | { "INIT" => "init", "PENDING" => "pend", "HOLD" => "hold", "ACTIVE" => "actv", "STOPPED" => "stop", "SUSPENDED" => "susp", "DONE" => "done", "FAILED" => "fail" } | ||
SHORT_LCM_STATES | = | { "PROLOG" => "prol", "BOOT" => "boot", "RUNNING" => "runn", "MIGRATE" => "migr", "SAVE_STOP" => "save", "SAVE_SUSPEND" => "save", "SAVE_MIGRATE" => "save", "PROLOG_MIGRATE"=> "migr", "PROLOG_RESUME" => "prol", "EPILOG_STOP" => "epil", "EPILOG" => "epil", "SHUTDOWN" => "shut", "CANCEL" => "shut", "FAILURE" => "fail", "CLEANUP" => "clea", "UNKNOWN" => "unkn" } | ||
MIGRATE_REASON | = | %w{NONE ERROR STOP_RESUME USER CANCEL} | ||
SHORT_MIGRATE_REASON | = | { "NONE" => "none", "ERROR" => "erro", "STOP_RESUME" => "stop", "USER" => "user", "CANCEL" => "canc" } |
Public class methods
Creates a VirtualMachine description with just its identifier this method should be used to create plain VirtualMachine objects. id the id of the vm
Example:
vnet = VirtualMachine.new(VirtualMachine.build_xml(3),rpc_client)
# File OpenNebula/VirtualMachine.rb, line 86 86: def VirtualMachine.build_xml(pe_id=nil) 87: if pe_id 88: vm_xml = "<VM><ID>#{pe_id}</ID></VM>" 89: else 90: vm_xml = "<VM></VM>" 91: end 92: 93: XMLElement.build_xml(vm_xml, 'VM') 94: end
# File OpenNebula/VirtualMachine.rb, line 96 96: def VirtualMachine.get_reason(reason) 97: reason=MIGRATE_REASON[reason.to_i] 98: reason_str=SHORT_MIGRATE_REASON[reason] 99: 100: reason_str 101: end
Class constructor
# File OpenNebula/VirtualMachine.rb, line 106 106: def initialize(xml, client) 107: super(xml,client) 108: 109: @element_name = "VM" 110: @client = client 111: end
Public instance methods
Allocates a new VirtualMachine in OpenNebula
description A string containing the template of the VirtualMachine.
# File OpenNebula/VirtualMachine.rb, line 125 125: def allocate(description) 126: super(VM_METHODS[:allocate],description) 127: end
Cancels a running VM
# File OpenNebula/VirtualMachine.rb, line 148 148: def cancel 149: action('cancel') 150: end
Initiates the instance of the VM on the target host.
host_id The host id (hid) of the target host where the VM will be instantiated.
# File OpenNebula/VirtualMachine.rb, line 133 133: def deploy(host_id) 134: return Error.new('ID not defined') if !@pe_id 135: 136: rc = @client.call(VM_METHODS[:deploy], @pe_id, host_id.to_i) 137: rc = nil if !OpenNebula.is_error?(rc) 138: 139: return rc 140: end
Deletes a VM from the pool
# File OpenNebula/VirtualMachine.rb, line 178 178: def finalize 179: action('finalize') 180: end
Sets a VM to hold state, scheduler will not deploy it
# File OpenNebula/VirtualMachine.rb, line 153 153: def hold 154: action('hold') 155: end
Retrieves the information of the given VirtualMachine.
# File OpenNebula/VirtualMachine.rb, line 118 118: def info() 119: super(VM_METHODS[:info], 'VM') 120: end
Returns the LCM state of the VirtualMachine (numeric value)
# File OpenNebula/VirtualMachine.rb, line 242 242: def lcm_state 243: self['LCM_STATE'].to_i 244: end
Returns the LCM state of the VirtualMachine (string value)
# File OpenNebula/VirtualMachine.rb, line 247 247: def lcm_state_str 248: LCM_STATE[lcm_state] 249: end
Migrates a running VM to another host without downtime
# File OpenNebula/VirtualMachine.rb, line 203 203: def live_migrate(host_id) 204: return Error.new('ID not defined') if !@pe_id 205: 206: rc = @client.call(VM_METHODS[:migrate], @pe_id, host_id.to_i, true) 207: rc = nil if !OpenNebula.is_error?(rc) 208: 209: return rc 210: end
Saves a running VM and starts it again in the specified host
# File OpenNebula/VirtualMachine.rb, line 193 193: def migrate(host_id) 194: return Error.new('ID not defined') if !@pe_id 195: 196: rc = @client.call(VM_METHODS[:migrate], @pe_id, host_id.to_i, false) 197: rc = nil if !OpenNebula.is_error?(rc) 198: 199: return rc 200: end
Releases a VM from hold state
# File OpenNebula/VirtualMachine.rb, line 158 158: def release 159: action('release') 160: end
Forces a re-deployment of a VM in UNKNOWN or BOOT state
# File OpenNebula/VirtualMachine.rb, line 183 183: def restart 184: action('restart') 185: end
Resubmits a VM to PENDING state
# File OpenNebula/VirtualMachine.rb, line 188 188: def resubmit 189: action('resubmit') 190: end
Resumes the execution of a saved VM
# File OpenNebula/VirtualMachine.rb, line 173 173: def resume 174: action('resume') 175: end
Set the specified vm’s disk to be saved in a new image when the VirtualMachine shutdowns
disk_id ID of the disk to be saved
image_id ID of the new image where the disk will be saved
# File OpenNebula/VirtualMachine.rb, line 218 218: def save_as(disk_id, image_id) 219: return Error.new('ID not defined') if !@pe_id 220: 221: rc = @client.call(VM_METHODS[:savedisk], @pe_id, disk_id, image_id) 222: rc = nil if !OpenNebula.is_error?(rc) 223: 224: return rc 225: end
Shutdowns an already deployed VM
# File OpenNebula/VirtualMachine.rb, line 143 143: def shutdown 144: action('shutdown') 145: end
Returns the VM state of the VirtualMachine (numeric value)
# File OpenNebula/VirtualMachine.rb, line 232 232: def state 233: self['STATE'].to_i 234: end
Returns the VM state of the VirtualMachine (string value)
# File OpenNebula/VirtualMachine.rb, line 237 237: def state_str 238: VM_STATE[state] 239: end
Returns the short status string for the VirtualMachine
# File OpenNebula/VirtualMachine.rb, line 252 252: def status 253: short_state_str=SHORT_VM_STATES[state_str] 254: 255: if short_state_str=="actv" 256: short_state_str=SHORT_LCM_STATES[lcm_state_str] 257: end 258: 259: short_state_str 260: end
Stops a running VM
# File OpenNebula/VirtualMachine.rb, line 163 163: def stop 164: action('stop') 165: end
Saves a running VM
# File OpenNebula/VirtualMachine.rb, line 168 168: def suspend 169: action('suspend') 170: end